How to Design Custom Checkbox in Flutter with Getwidget
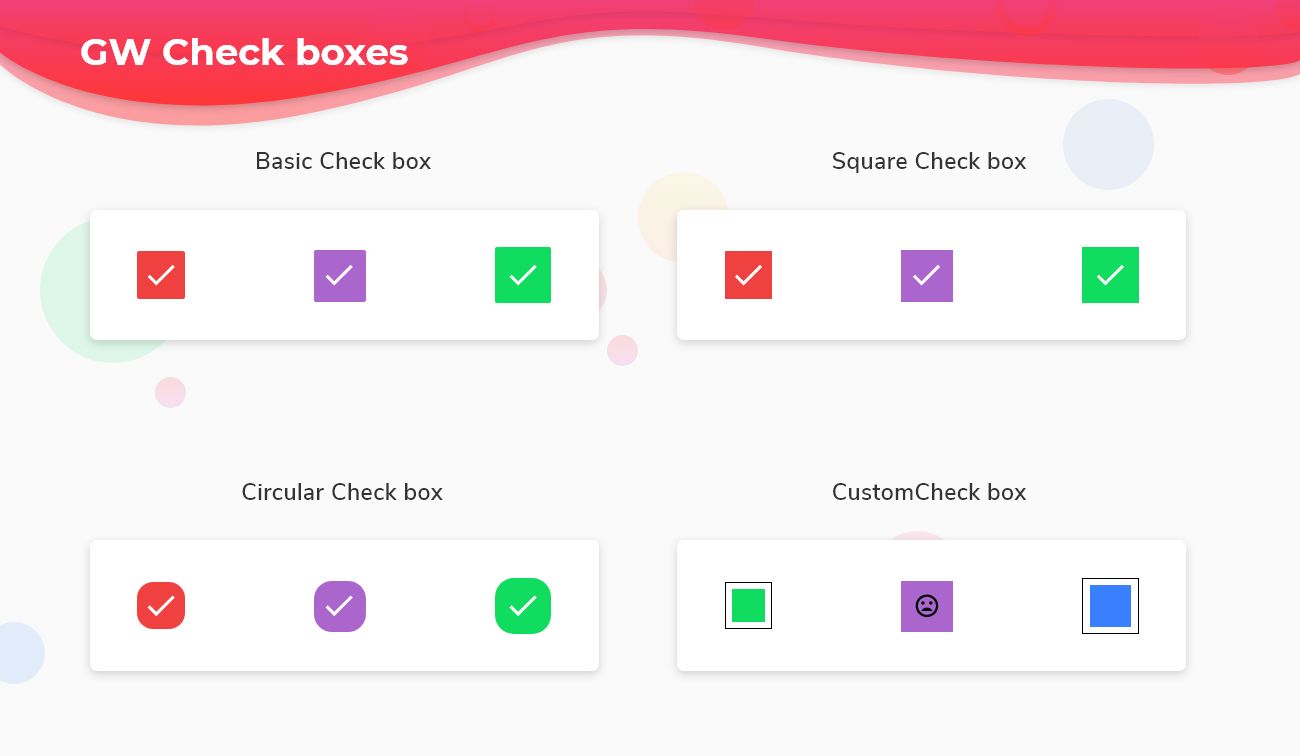
Flutter Checkbox is used when we have lists of options and the user can select any number of choices.
Flutter Checkbox is a GUI(Graphical User Interface) which allows the user to do a binary choice between yes and no options. This is alternatively a choice between possible outcomes i.e. if the user has a yes/no outcome there we can show the checkbox as checked/unchecked. Users can also use its customized properties to make it elegant.
In Flutter, we can define a Checkbox of widgets inside the scaffold. Therefore before adding a Checkbox of a widget, we need to define a Scaffold. When we creating a sample flutter app, it comes with the default scaffold.
GF Checkbox Widget for Flutter
It allows you to speed up your Flutter app development by 20-30%. It has almost all widget that allows you to enhance your Flutter UI design and speed up flutter development with fully customizable features.
Now here is i am going to talk about what is GetWidget Checkbox widget and how we implement this on Flutter app to build awesome Flutter Checkbox widget for an app.
So, are you ready to make use of this widget package in the Flutter application? If so, then let's quickly jump into the usage and the ways a Checkbox can be modified and used to make user-friendly apps. Here I am going to use an open-source UI Library known as GetWidget to build this Checkbox widget in Flutter.
How to Get Started
Now here is the guide about how we should start developing GFCheckbox Widget with the use of GetWidget UI Library.
Getting started will guide you on how to start building a beautiful flutter application with GetWidget UI library. You have to install the package from pub.dev, import the package in your Flutter project.
Install Package from pub.dev :
https://pub.dev/packages/getwidget
Import full package:
import 'package:getwidget/getwidget.dart';
Note: dependencies: getwidget: ^ 4.0.0
Keep playing with the pre-built UI components.
GFCheckBox is a Flutter Checkbox that is a material widget that allows users to select one option from the set of given options. The user can select one or multiple at a time depending on the situation. The checkbox is a binary value with yes and no. The value is checked if it is yes and unchecked if it is no.
Let us now see the different types of GFCheckBox in the below section
Flutter Basic Checkbox
This is a basic or standard type of checkbox. It has a slight border radius around it. By using the below code we can design a basic Checkbox.
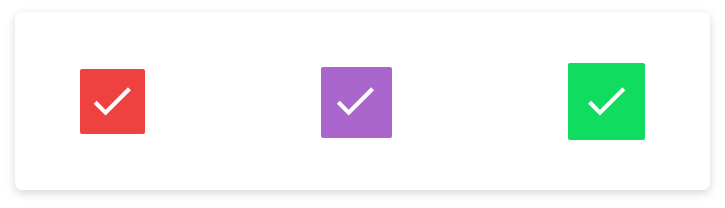
bool isChecked = false;
GFCard(
content: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
GFCheckbox(
activeBgColor: Colors.red,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: null,
),
GFCheckbox(
activeBgColor: Colors.blue,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: null,
),
GFCheckbox(
size: GFSize.SMALL,
activeBgColor: Colors.green,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: null,
),
],
),
),
Flutter Simple checkbox
Flutter Square Checkbox
This is a square type of checkbox. It doesn't have any border radius. We use GFCheckboxType.square to make it of type square. Use the below code to make a square Checkbox.

bool isChecked = true;
GFCard(
content: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
GFCheckbox(
size: GFSize.LARGE,
activeBgColor: Colors.red,
type: GFCheckboxType.square,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: null,
),
GFCheckbox(
type: GFCheckboxType.square,
activeBgColor: Colors.blue,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: null,
),
GFCheckbox(
size: GFSize.SMALL,
activeBgColor: Colors.green,
type: GFCheckboxType.square,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: null,
),
],
),
),
Flutter Square checkbox widget
Flutter Circular Checkbox
This is a circular type checkbox. The shape is of a circle or round as shown in the below image. Add type GFCheckboxType.circle to make it a circle.
Use the below example to show the Flutter circular Checkbox.

bool isChecked = false;
GFCard(
content: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
GFCheckbox(
size: GFSize.LARGE,
activeBgColor: Colors.red,
type: GFCheckboxType.circle,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: null,
),
GFCheckbox(
type: GFCheckboxType.circle,
activeBgColor: Colors.blue,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
),
GFCheckbox(
activeBgColor: Colors.green,
size: GFSize.MEDIUM,
type: GFCheckboxType.circle,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: null,
),
],
),
),
Flutter Circular Checkbox Widget
Flutter Custom Checkbox Widget With Icon
The Custom CheckBox can have an icon or background image as the check value shown in the image below. We can customize the widget by using its property. Use the below example to make a custom Checkbox.

bool isChecked = true;
GFCard(
content: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
GFCheckbox(
size: GFSize.LARGE,
type: GFCheckboxType.custom,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: null,
),
GFCheckbox(
type: GFCheckboxType.square,
activeBgColor: Colors.purple,
activeIcon: Icon(Icons.sentiment_satisfied),
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
inactiveIcon: Icon(Icons.sentiment_dissatisfied),
),
GFCheckbox(
size: GFSize.SMALL,
type: GFCheckboxType.custom,
onChanged: (value) {
setState(() {
isChecked = value;
});
},
value: isChecked,
custombgColor: Colors.blue,
),
],
),
),
Flutter Custom Checkbox Widget
Flutter Checkbox Widget Custom Properties
The look and feel of the Flutter Checkbox can be customized using the GFCheckbox properties.
type | type of [GFCheckboxType] which is of four type is basic, square, circular and custom |
size | type of [double] which is GFSize ie, small, medium and large and can use any double value |
activeBgColor | type of [Color] used to change the backgroundColor of the active checkbox |
inactiveBgColor | type of [Color] used to change the backgroundColor of the inactive checkbox |
activeBorderColor | type of [Color] used to change the border color of the active checkbox |
inactiveBorderColor | type of [Color] used to change the border color of the inactive checkbox |
onChanged | called when the user checks or unchecks the checkbox. |
value | used to set the current state of the checkbox |
activeIcon | type of [Widget] used to change the checkbox's active icon |
inactiveIcon | type of [Widget] used to change the checkbox's inactive icon |
customBgColor | type of [Color] used to change the background color of the custom active checkbox only |
autofocus | on true state this widget will be selected as the initial focus when no other node in its scope is currently focused |
focusNode | an optional focus node to use as the focus node for this widget. |
Can we change the tick mark?
Yes, we can change the tick mark by using its customizable property.
How to change the size of the CheckBox
The size can be changed using the size property and the GFCheckBox has small, medium, and large by default. The user can also change according to the need by giving the double value.
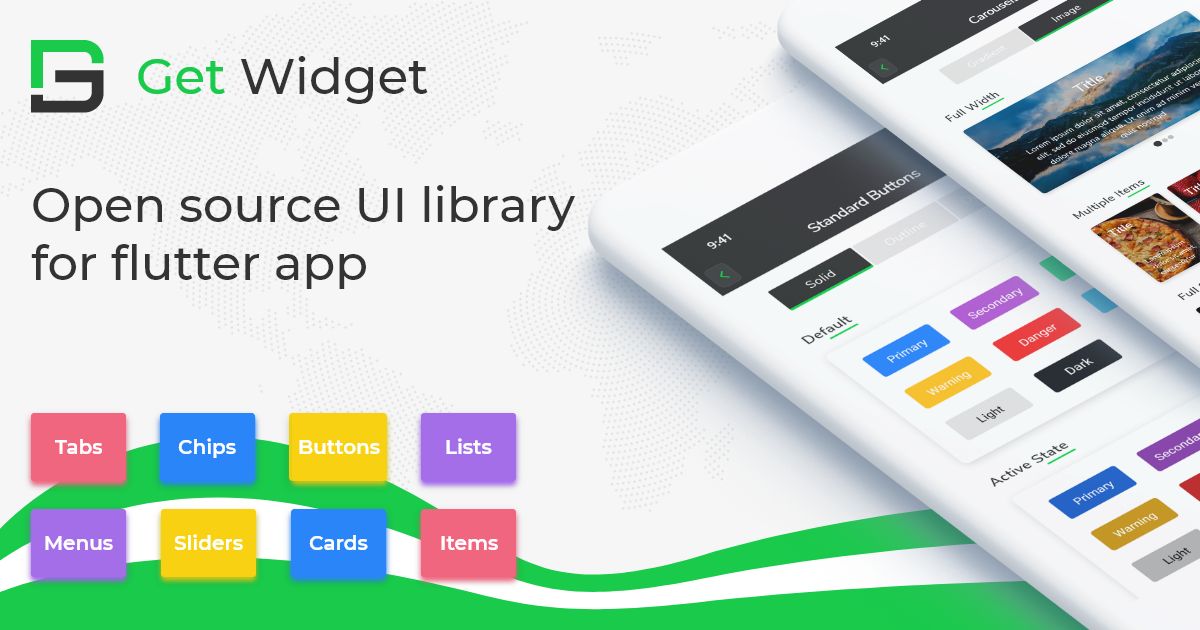
GitHub Repository: Please do appreciate our work through Github start
Conclusion:
Here we discuss, what Flutter CheckBox Widget is? And how we can use and implement it into our Flutter app through the GetWidget CheckBox component. Here, there are options to customize and use other types of CheckBox.
About Our Team:
We have been working on Flutter since Flutter launched in beta version in 2017. And our team have been putting hundreds and hundreds of hour into the experiment and implementation of Flutter. As well as after the successful delivery of enterprise and SAAS applications that have been used by more than 500+ businesses around the 119+ countries. Now we are in love with Flutter development and we are very passionate about Flutter development. Now it is something we are trying to give a small contribution to the Flutter Dev Community. If you need assistance for your next app development project you can hire flutter developer from the GetWidget team