Flutter Radio Button - A graphically control element that allows the user to choose one among a predefined list.
You can create a custom radio button with many custom properties like a Square radio button, ios type radio button, and many more with example code.
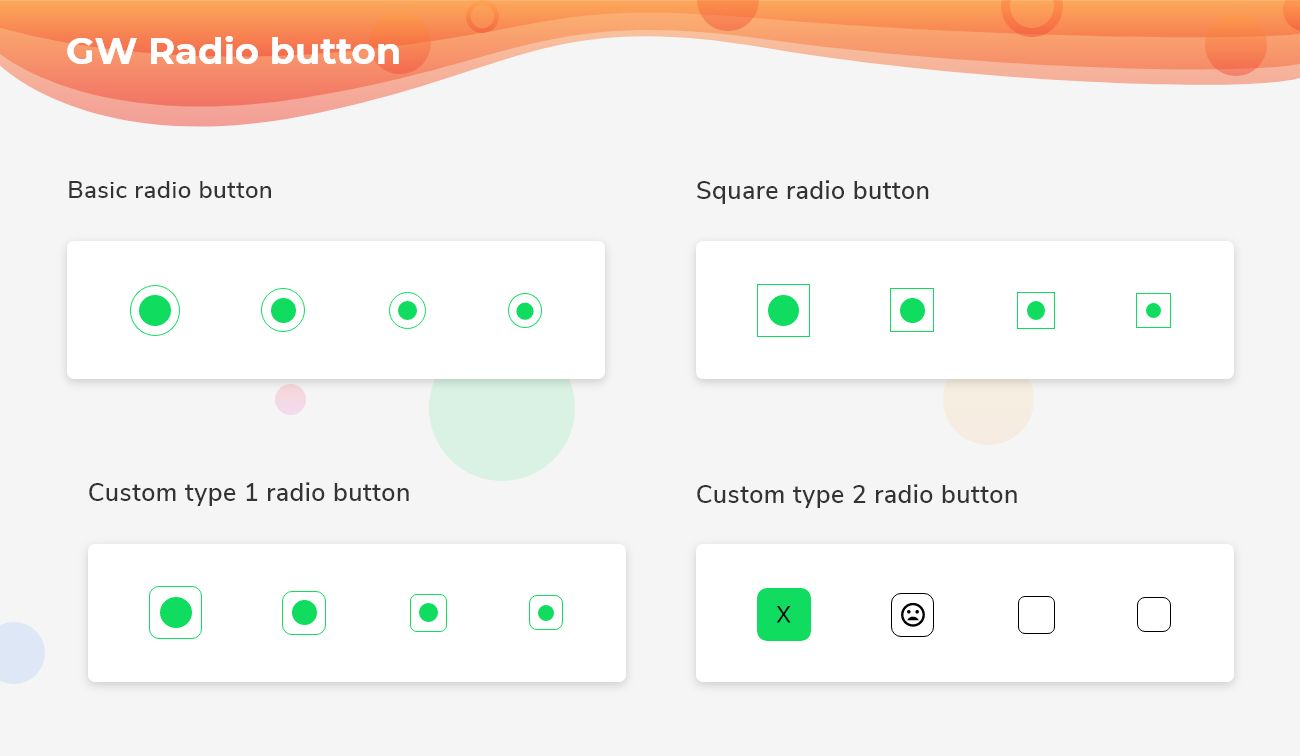
Flutter Radio Button allows users to make a choice among a list. We are likely to use this where you have to pick only one option among a list.
Flutter Radio is a GUI(Graphical User Interface) which allows the user to do a binary choice. This is alternatively a choice among possible outcomes i.e. if the user has a yes/no outcome there we can show Radio as checked/unchecked. Radio can be checked only once. Users can also use its customized properties to make it elegant. This is basically a rounded border with having a solid circle inside it , when it is checked otherwise it's just a blank rounded border.
In Flutter, we can define the Radio of widgets inside the scaffold. Therefore before adding a Radio of a widget we need to define a Scaffold. When we creating a sample flutter app, it comes with the default scaffold.
Radio Button Widget in Flutter With Example
I am going to talk about what is GetWidget Radio widget is and how we implement this on the Flutter app to build an awesome Flutter Radio widget for an app.
So, are you ready to make use of this widget package in the Flutter application? If so, then let's quickly jump into the usage and the ways a Radio can be modified and used to make user-friendly apps. Here I am going to use an open-source flutter UI Library known as GetWidget to build this Radio option widget in Flutter.
How to Start:
Now here is the guide about how we should start developing GFRadio Widget with the use of GetWidget UI Library.
Getting started will guide you on how to start building a beautiful flutter application with GetWidget UI library. You have to install the package from pub.dev, import the package in your Flutter project.
Install Package from pub.dev :
https://pub.dev/packages/getwidget
Import full package:
import 'package:getwidget/getwidget.dart';
Note: dependencies: getwidget: ^ 4.0.0
Keep playing with the pre-built UI components.
GFRadio Button is a Flutter Radio Button that is a material widget that has a binary value of yes or no. The user can select only one value at a time in the given set of options. The checked value will be a yes and the unchecked value will be a no.
Also Read - How to Choose the Right Flutter App Development Company: A Comprehensive Guide
Let us now see the different types of GFRadio buttons in the below section
Basic Flutter Radio Button Example Code
This is a basic type of radio button that is circular in shape and has a dot for the checked and blank for the unchecked options.
Let us see the radio button in flutter examples below.
By using the below code we can design a basic Radio button in GFRadio.
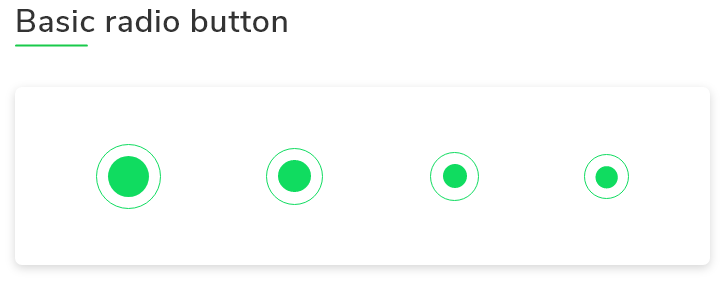
int groupValue = 0;
GFCard(
content: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
GFRadio(
size: GFSize.LARGE,
activeBorderColor: Colors.green,
value: 0,
groupValue: groupValue,
onChanged: (val) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
radioColor: Colors.green,
),
GFRadio(
size: GFSize.MEDIUM,
value: 1,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
activeBorderColor: Colors.green,
radioColor: Colors.green,
),
GFRadio(
size: GFSize.SMALL,
value: 2,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
activeBorderColor: Colors.green,
radioColor: Colors.green,
),
GFRadio(
size: 20,
value: 3,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
activeBorderColor: Colors.green,
radioColor: Colors.green,
)
],
)),
Basic Custom Flutter radio option button
Flutter Square Radio button design with example code
This is a square type of Radio. Here the border is of type square but the inside child is a solid circle. Use the below code to make a custom square Radio Button.
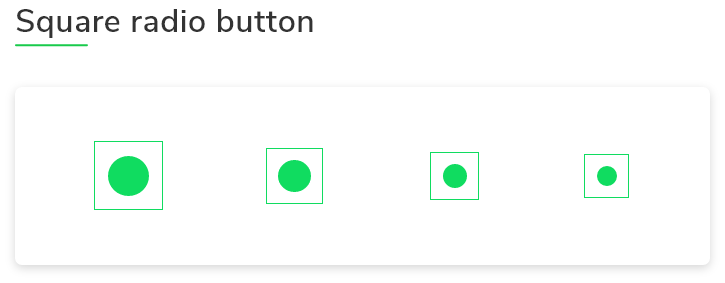
int groupValue = 0;
GFCard(
content: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
GFRadio(
type: GFRadioType.square,
size: GFSize.LARGE,
value: 1,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
activeBorderColor: Colors.green,
radioColor: Colors.green,
),
GFRadio(
type: GFRadioType.square,
size: GFSize.MEDIUM,
value: 2,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
activeBorderColor: Colors.green,
radioColor: Colors.green,
),
GFRadio(
type: GFRadioType.square,
size: GFSize.SMALL,
value: 3,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
activeBorderColor: Colors.green,
radioColor: Colors.green,
),
GFRadio(
type: GFRadioType.square,
size: 20,
value: 7,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
activeBorderColor: Colors.green,
radioColor: Colors.green,
)
],
)),
Flutter Square Radio button with example Code snippet
Custom Flutter Radio Button Design with example code
Custom radio button is a type of radio button where-in, the user can cutomize the radio button according to the needs of the application.This is a custom type of Radio. It can have icons as the checked value according to the requirement to make the look of the radio more attractive to the user. We can customize the widget by using its property. Use the below example to make a custom Radio.
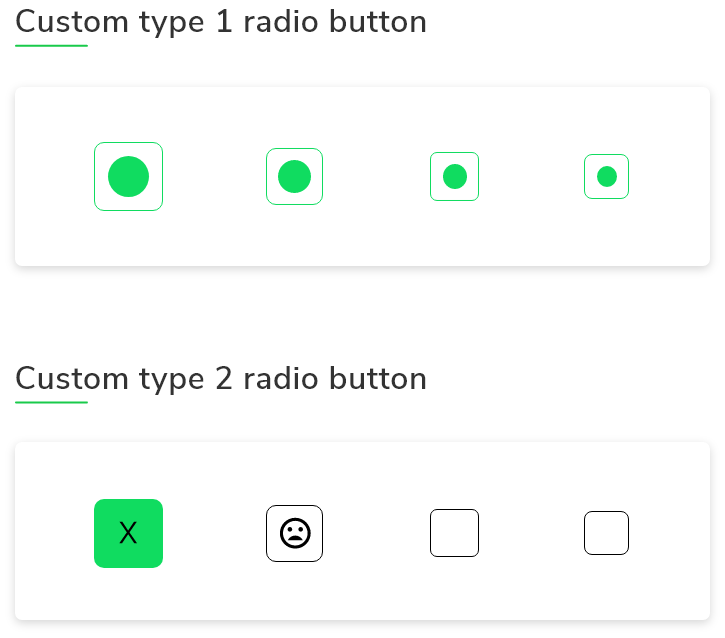
int groupValue = 0;
GFCard(
content: Row(
mainAxisAlignment: MainAxisAlignment.spaceBetween,
children: <Widget>[
GFRadio(
type: GFRadioType.custom,
activeIcon: Icon(Icons.check),
radioColor: Colors.red,
size: GFSize.LARGE,
activeBgColor: Colors.green,
inactiveBorderColor: Colors.black,
activeBorderColor: Colors.green,
value: 1,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
),
GFRadio(
type: GFRadioType.custom,
activeIcon: Icon(Icons.sentiment_satisfied),
size: GFSize.MEDIUM,
value: 2,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: Icon(Icons.sentiment_dissatisfied),
customBgColor: Colors.red,
activeBgColor: Colors.green,
activeBorderColor: Colors.green,
),
GFRadio(
type: GFRadioType.blunt,
size: GFSize.SMALL,
value: 3,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
customBgColor: Colors.green,
activeBorderColor: Colors.green,
),
GFRadio(
type: GFRadioType.blunt,
size: 25,
value: 4,
groupValue: groupValue,
onChanged: (value) {
setState(() {
groupValue = value;
});
},
inactiveIcon: null,
activeBorderColor: Colors.green,
customBgColor: Colors.green,
),
],
)),
Custom Flutter Radio button design with example code snippet
Flutter Radio Widget Custom Properties
The look and feel of the Flutter radio Button Widget can be customized using the GFRadio properties.
type | type of [GFRadioType] which is of four type is basic, sqaure, circular and custom |
size | type of [double] which is GFSize ie, small, medium and large and can use any double value |
radioColor | type pf [Color] used to change the checkcolor when the radio button is active |
activeBgColor | type of [Color] used to change the backgroundColor of the active radio button |
inactiveBgColor | type of [Color] used to change the backgroundColor of the inactive radio button |
activeBorderColor | type of [Color] used to change the border color of the active radio button |
inactiveBorderColor | type of [Color] used to change the border color of the inactive radio button |
onChanged | Called when the user checks or unchecks the radio button |
activeIcon | type of Widget used to change the radio button's active icon |
inactiveIcon | type of [Widget] used to change the radio button's inactive icon |
customBgColor | type of [Color] used to change the background color of the custom active radio button only |
autofocus | on true state this widget will be selected as the initial focus when no other node in its scope is currently focused |
focusNode | an optional focus node to use as the focus node for this widget. |
value | The value represented by this radio button. |
groupValue | The currently selected value for a group of radio buttons. Radio button is considered selected if its [value] matches the [groupValue]. |
toggleable | sets the radio value |
How to change alignment of radio button in flutter?
In Getwidget Radio button, we can easily change the position or alignment of the radio button to left or right side of the page. It is achieved through a property called "position" where-in we have to define the button position to be start or end.
What is the difference between radio buttons and checkboxes?
In simple terms, the main difference b/w radio buttons and checkboxes is that, in radio buttons only one unique value is selected from a list of items or values whereas in checkboxes multiple values can be selected in the list of items.
Can we change the solid circle of the radio?
Yes, we can change the solid circle by using its customizable property.
How to change the size of the GFRadio
The size can be changed using the size property and the GFRadio has small, medium, and large by default. The user can also change according to the need by giving the double value.
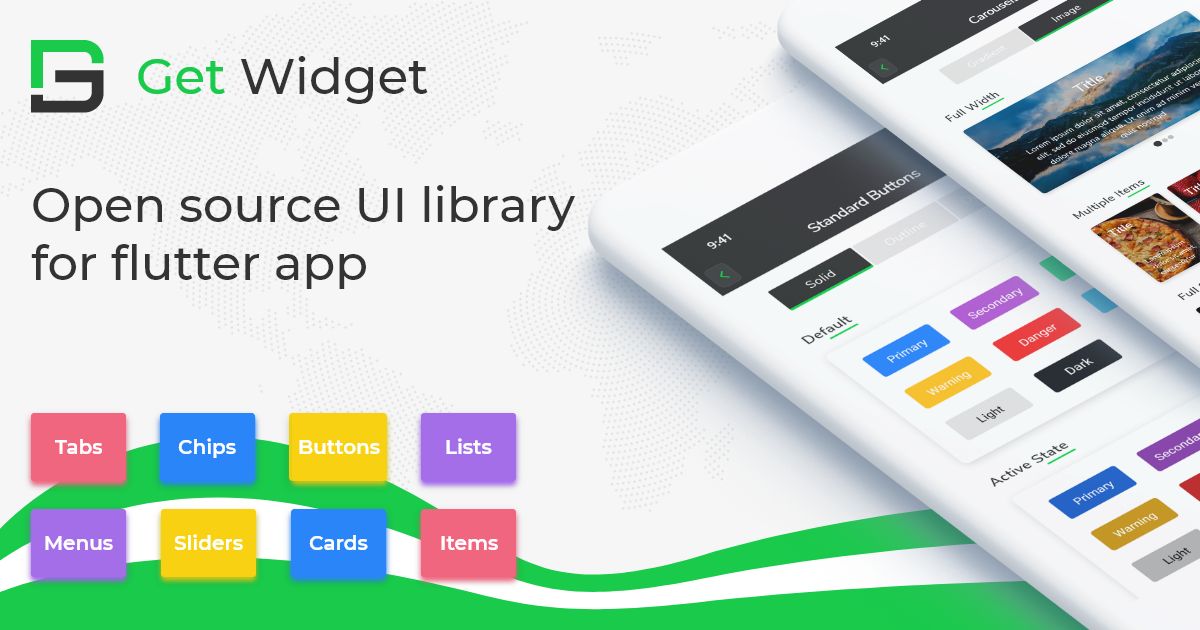
GitHub Repository: Please do appreciate our work through Github start
Conclusion:
Here we discuss, what Flutter Radio Widget is? And how we can use and implement it into our Flutter app through the GetWidget Radio component. Here, there are options to customize and use other types of Radio buttons.
About Our Team:
We have been working on Flutter since Flutter launched in beta version in 2017. And our team have been putting hundreds and hundreds of hour to experiment and implementation of Flutter. As well as after successful delivery of enterprise and SAAS applications that have been used by more than 500+ businesses around the 119+ countries. Now we are in love with Flutter development and we are very passionate about Flutter development. Now it is something we are trying to give a small contribution to the Flutter Dev Community. If you need assistance for your next app development project you can hire flutter developer from the GetWidget team