How to Implement Badges in a Flutter App using the 'Badges' Package?
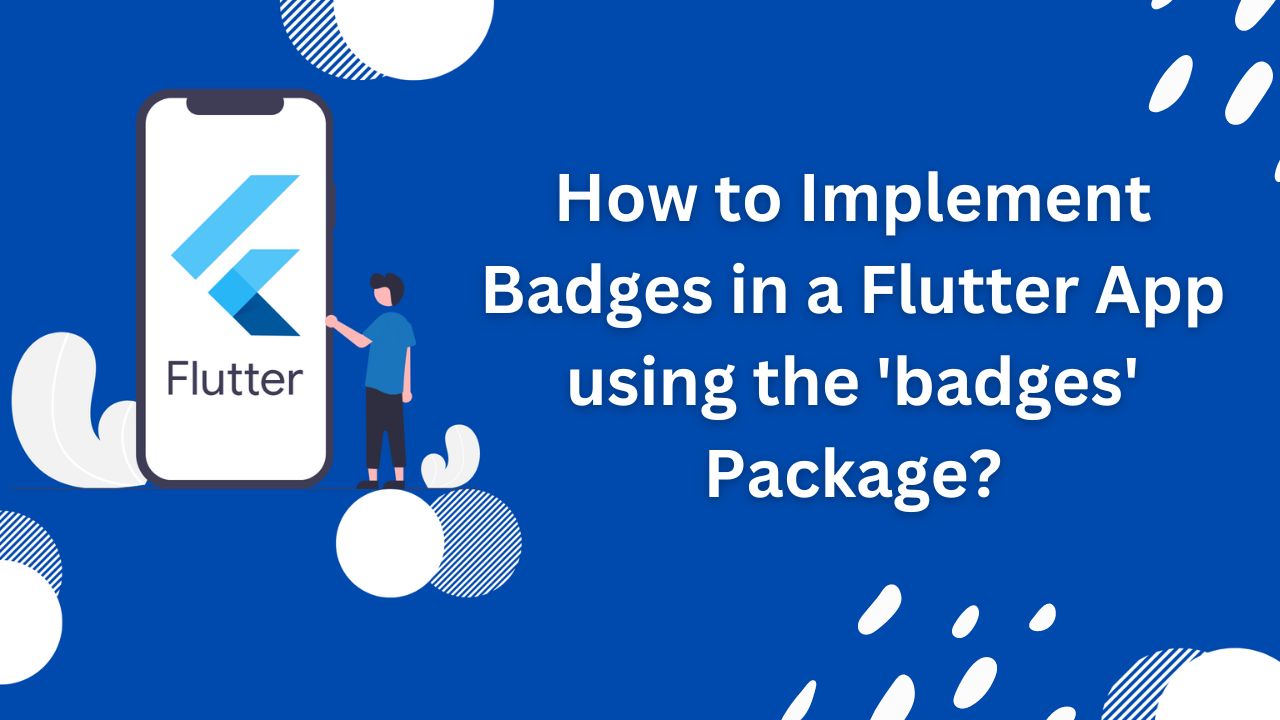
In the fast-paced world of mobile app development, user engagement and retention are paramount to the success of an application. One effective way to keep users informed and engaged is by utilizing badges, a popular visual indicator that notifies users about new messages, updates, or notifications within a mobile app. In the context of Flutter app development, integrating badges seamlessly into the user interface can significantly enhance the overall user experience.
Importance of Badges in Flutter Apps
Badges serve as unobtrusive yet attention-grabbing markers that alert users to important events or updates within an app. Whether it's a pending message, a new feature, or a critical notification, badges provide a convenient and intuitive way to communicate these events to users without disrupting their interaction with the app.
In a Flutter app, badges can be strategically applied to various UI elements, such as Flutter icons, Flutter buttons, or Flutter app bars, offering a subtle yet effective means of conveying information to users. By leveraging the 'badges' package in Flutter, developers can seamlessly integrate these visual cues, enhancing the app's usability and ensuring that users stay informed and engaged.
Throughout this blog post, we'll explore the process of implementing badges in a Flutter app using the 'badges' package, covering everything from setup and customization to best practices for handling badge count updates. By the end of this guide, you'll be well-equipped to leverage badges effectively and elevate the user experience within your Flutter app.
With the groundwork laid, let's dive into the world of badges in Flutter app development and learn how to seamlessly integrate these visual cues to enhance user engagement and interaction.
What are Badges in Flutter?
In the context of a Flutter app, badges are visual indicators that are typically used to convey specific information to the user. These indicators are often represented as small, circular elements containing numerical values or custom icons, and they are commonly overlaid on top of other UI elements such as icons, buttons, or app bars.
Significance of Using Badges in Flutter Apps
- Enhanced User Engagement: Badges play a crucial role in capturing and retaining users' attention. By providing visual cues for new messages, notifications, or updates, badges prompt users to interact with the app, thereby increasing overall user engagement.
- Seamless Information Conveyance: In a cluttered digital environment, users may overlook important updates or notifications. Badges cut through this digital noise by succinctly communicating the presence of new information or events, ensuring that users remain informed without the need for intrusive alerts.
- Intuitive User Experience: When implemented thoughtfully, badges contribute to an intuitive and user-friendly interface. They enable users to quickly identify areas of interest within the app, fostering a seamless and efficient user experience.
- Retention and Return Visits: By effectively using badges to signify new content or updates, Flutter app developers can encourage users to return to the app, thereby improving user retention and fostering a sense of ongoing engagement.
Overview of the 'badges' Package
Introducing the 'badges' package for Flutter
The 'badges' package for Flutter is a versatile and user-friendly solution for integrating badges into Flutter applications. This package provides developers with a robust set of tools and components to effortlessly incorporate badges into their app's user interface, allowing for seamless communication of new messages, updates, or notifications to users.
Features and Capabilities of the 'badges' Package
Customizable Badges: The 'badges' package empowers developers to create badges with custom styles, including various shapes, colors, and sizes, ensuring that badges align with the app's visual identity and design.
Flexible Integration: With the 'badges' package, badges can be seamlessly integrated into different UI elements, such as icons, buttons, or app bars, offering flexibility in placement and usage within the app's interface.
Dynamic Content: Developers can easily update the content of badges, such as the numerical count or the presence of new notifications, to reflect real-time changes within the app, ensuring that users receive accurate and up-to-date information.
Accessibility Support: The 'badges' package is designed to maintain accessibility standards, ensuring that badges remain perceivable and operable for users with diverse needs and abilities.
Animation and Interactivity: The package provides support for animations and interactivity, allowing developers to incorporate engaging visual effects and user interactions to further enhance the badge experience.
Popularity of the 'badges' Package in Flutter App Development
The 'badges' package has gained popularity among Flutter developers due to several compelling reasons:
Simplicity and Ease of Use: The package offers a straightforward and intuitive API, making it accessible to developers of varying experience levels.
Community Support: With an active community and ongoing development, the 'badges' package benefits from continuous improvements, updates, and a wealth of resources and examples shared by the Flutter community.
Consistent Performance: The 'badges' package is known for its reliability and consistent performance, ensuring that badges function seamlessly across different devices and screen sizes.
By leveraging the 'badges' package, Flutter developers can efficiently implement badges within their apps, enriching the user experience and fostering effective communication of important app events to users.
Setting Up the Flutter Project
Creating a New Flutter Project or Using an Existing One
If you are starting a new Flutter project:
- Open a terminal or command prompt.
- Navigate to the directory where you want to create the project.
- Run the following command to create a new Flutter project: flutter create your_project_name
- Once the project is created, navigate to the project directory using: cd your_project_name
If you are using an existing Flutter project, ensure that you are in the root directory of your project.
Adding the 'badges' Package to the Project's Dependencies
To integrate the 'badges' package into your Flutter project, follow these
1. Open the pubspec.yaml file in your Flutter project.
2. Locate the dependencies section within the pubspec.yaml file.
3. Add the 'badges' package as a dependency under the dependencies section:
dependencies:
flutter:
sdk: flutter
badges: ^1.1.0 # Replace with the latest version of the badges package
- Save the pubspec.yaml file.
- Run the following command in the terminal to fetch and install the 'badges' package: flutter pub get
By following these steps, you will have successfully added the 'badges' package to your Flutter project's dependencies, allowing you to begin utilizing the package to implement flutter badges within your app.
Implementing Badges for Widgets
Adding Badges to Icons
To add badges to icons in Flutter using the 'badges' package, follow these steps:
1. Import the necessary packages at the top of your Dart file:
import 'package:flutter/material.dart';
import 'package:badges/badges.dart';
2. Use the Badge widget to wrap the icon widget and specify the badge content and appearance:
Badge(
badgeContent: Text('3'), // Replace '3' with the actual badge count
child: Icon(Icons.notifications),
)
Adding Badges to Buttons
To incorporate badges with buttons, follow these steps:1. Wrap the button widget with the Badge widget and configure the badge properties:
Badge(
badgeContent: Text('New'), // Replace 'New' with any custom badge content
child: ElevatedButton(
onPressed: () {
// Button press logic
},
child: Text('Notifications'),
),
)
Adding Badges to App Bars
When adding badges to app bars, you can follow a similar approach:1. Wrap the app bar action with the Badge widget to display the badge:
AppBar(
actions: <Widget>[
Badge(
badgeContent: Text('5'), // Replace '5' with the actual badge count
position: BadgePosition.topEnd(top: 0, end: 3),
child: IconButton(
icon: Icon(Icons.notifications),
onPressed: () {
// Notification action logic
},
),
),
],
)
By following these examples, you can effectively integrate badges into various Flutter widgets, enhancing the visual presentation of new messages, updates, or notifications within your app.
Customizing Badges
In Flutter using the 'badges' package, you can customize badges to match your app's design by adjusting various properties such as colors, sizes, and positions. Below are some customization options along with code snippets and visual examples:
Changing Badge Colors
You can change the background and text colors of the badge to align with your app's theme. Here's an example of customizing badge colors:
Badge(
badgeColor: Colors.red, // Specify the background color of the badge
badgeContent: Text('New', style: TextStyle(color: Colors.white)), // Custom text color
child: IconButton(
icon: Icon(Icons.notifications),
onPressed: () {
// Notification action logic
},
),
)
Adjusting Badge Sizes
You can modify the size of the badge to ensure it fits well with the associated widget. Here's how you can adjust the badge size:
Badge(
padding: EdgeInsets.all(8.0), // Add padding to increase the badge size
badgeContent: Text('3'),
child: Icon(Icons.notifications),
)
Changing Badge Positions
You have the flexibility to position the badge relative to its parent widget. Here's an example of changing the badge position:
Badge(
badgeContent: Text('5'),
position: BadgePosition.topEnd(top: 0, end: 3), // Position the badge at the top end
child: IconButton(
icon: Icon(Icons.notifications),
onPressed: () {
// Notification action logic
},
),
)
By leveraging these customization options, you can tailor the appearance of badges within your Flutter app to seamlessly integrate with your app's design and user interface.
Handling Badge Count Updates
In Flutter, dynamically updating badge counts based on app events, notifications, or user interactions is essential for providing real-time feedback to users. Here's how you can manage badge counts and ensure a seamless user experience:
Updating Badge Counts Dynamically
To update badge counts dynamically, you can utilize state management techniques such as setState for updating the badge content based on app events or user interactions. For example, if a new notification is received, you can update the badge count as follows:
int _notificationCount = 0;
// Update the badge count based on new notifications
void updateNotificationCount() {
setState(() {
_notificationCount++; // Increment the notification count
});
}
Best Practices for Managing Badge Counts
To ensure a seamless user experience, consider the following best practices for managing badge counts:
- Real-time Updates: Update badge counts in real-time as new events or notifications occur within the app.
- Clearing Badge Counts: Provide a mechanism to reset or clear badge counts when users view the associated content, ensuring that the badge accurately reflects unread items.
- Consistent Feedback: Use badge counts judiciously and provide consistent feedback to users, avoiding excessive use of badges that may lead to user fatigue.
- Accessibility: Ensure that badge counts are accessible to all users, including those with visual impairments, by providing alternative methods of notification.
By following these best practices and updating badge counts dynamically based on relevant app events, you can enhance user engagement and provide a seamless experience within your Flutter app.
Testing and Debugging Badge Implementations
Importance of Testing
Testing badge implementations in different scenarios is crucial to ensure a consistent and reliable user experience. Here's why testing is important:
User Experience: Testing helps verify that badge displays and behavior align with user expectations, enhancing the overall user experience.
Functional Integrity: Thorough testing ensures that badge counts update correctly and badges appear as intended across various app states and user interactions.
Compatibility: Testing in different scenarios helps identify any compatibility issues with different devices, screen sizes, and orientations.
Tips for Debugging
When debugging common issues related to badge display and behavior, consider the following tips:
Check Widget Hierarchy: Verify that the badge widget is correctly nested within the target widget hierarchy to ensure proper display.
State Management: Review the state management logic to ensure that badge counts are updated accurately based on app events or user interactions.
Visual Inspection: Inspect the UI to identify any layout or positioning issues that may affect badge visibility or alignment.
Testing Edge Cases: Test edge cases such as zero badge counts, maximum count display, and long text to ensure that badges handle these scenarios gracefully.
Device Emulation: Test badge behavior across different device emulators to identify any display inconsistencies on various screen sizes and resolutions.
Embrace the 'badges' Package
By leveraging the 'badges' package, Flutter app developers can add an intuitive and visually engaging way to display notifications, alerts, or other indicators within their apps. The package's flexibility and customization options empower developers to tailor badge displays to match their app's design and provide real-time feedback to users.
We encourage readers to explore the 'badges' package and integrate it into their Flutter projects to elevate the user interface and user experience, ultimately enhancing user engagement and interaction within their apps.