A Flutter search bar is an input field that is used to get the needed information from a collection of data through typing the words & keys related to that. Search bars are the most commonly used component in online shopping apps to find the stuff from a collection of stuff. The search bar is very much helpful to the user in such cases. Not only in shopping apps, but the search bar is also used everywhere having some dynamic information.
In technical terms there are 2 commonly search algorithms:
Linear Search
Linear search is a very simple search algorithm. In Linear search, we search an element or the value in a given array by traversing the array from the starting, till the desired element or value is found.
Binary Search
Binary Search is useful when there is a large number of elements in an array and they are sorted. So a necessary condition for Binary search to work is that the array should be sorted.
Flutter Search Bar with GFWidget
GF SearchBar is a GetWidget component that is used to build a flutter search bar in a flutter project. It represents a text field that can be used to search through a group. Flutter SearchBar can be used inside the app bar or in any page component wherever needed. It is very easy & simple to customize the GF SearchBar.
searchList: List of [text] or [widget] reference for users
overlaySearchListItemBuilder: Defines how the [searchList] items look like in overlayContainer
hideSearchBoxWhenItemSelected: If true, it will hide the [searchBox]
overlaySearchListHeight: Defines the height of [searchList] overlay container
searchQueryBuilder: Can search and filter the [searchList]
and much more property customization can be done.
How to Get Started
If you are new with GetWidget then you should go ahead with Getting started will guide you on how to start building a beautiful flutter application with the GetWidget UI library. You have to install the package from pub.dev, import the package in your Flutter project.
Install Package from pub.dev :
https://pub.dev/packages/getwidget
Import full package:
import ‘package:getwidget/getwidget.dart’;
Note: dependencies: getwidget: ^4.0.0
Keep playing with the pre-built UI components.
GFSearchBar is a search bar input field where the user presses some keys in order to get the relevant options from the list in the search bar that is provided. The below example shows a simple search bar and the result of the code is as shown in the image below.
import 'package:getwidget/getwidget.dart';
List list = [
"Flutter",
"Angular",
"Node js",
];
GFSearchBar(
searchList: list,
searchQueryBuilder: (query, list) => list
.where((item) {
return item!.toString().toLowerCase().contains(query.toLowerCase());
})
.toList(),
overlaySearchListItemBuilder: (dynamic item) => Container(
padding: const EdgeInsets.all(8),
child: Text(
item,
style: const TextStyle(fontSize: 18),
),
),
onItemSelected: (dynamic item) {
setState(() {
print('$item');
});
}),
GF Search Bar Widget Custom Properties
The look and feel of the GFSearhBar can be customized accordingly by using the below-listed properties.
Name | Description |
---|---|
searchList | List of [text] or [widget] reference for users |
overlaySearchListItemBuilder | defines how the [searchList] items look like in overlayContainer |
hideSearchBoxWhenItemSelected | if true, it will hide the [searchBox] |
overlaySearchListHeight | defines the height of [searchList] overlay container |
searchQueryBuilder | can search and filter the [searchList] |
noItemsFoundWidget | displays the [widget] when the search item failed |
onItemSelected | defines what to do with onSelect [SearchList] item |
searchBoxInputDecoration | defines the input decoration of [searchBox] |
Flutter Search Bar inside the AppBar
The GFSearchar can be used inside the GFAppbar that does the searching operation just like a normal search but with minimum lines of code in the appbar. If the search bar is true
then it displays a search bar text field with leading, trailing options.

The below code shows how a search bar should be passed in the appbar code.
import 'package:getwidget/getwidget.dart';
GFAppBar(
leading: GFIconButton(
icon: Icon(
Icons.message,
color: Colors.white,
),
onPressed: () {},
type: GFButtonType.transparent,
),
searchBar: true,
title: Text("GF Appbar"),
actions: [
GFIconButton(
icon: Icon(
Icons.favorite,
color: Colors.white,
),
onPressed: () {},
type: GFButtonType.transparent,
),
],
),
Why use GF Flutter Search Bar?
You will get a pre-designed Search Bar that can be used for searching data in your app. It is very easy to customize & will save your time of development.
Can we customize GF Search Bar?
Yes, You can customize the shape, border, position of the Search Bar according to need. Some custom properties are :
searchList
overlaySearchListItemBuilder
hideSearchBoxWhenItemSelected
overlaySearchListHeight
searchBoxInputDecoration
Where can we use GF Search Bar?
GF Search Bar can be used anywhere in your application and in the appbar as well.
Do you update the component in the future?
GetFlutter team is very much passionate about the up-gradation of technology and its related modules. The library & components get updated from time to time.
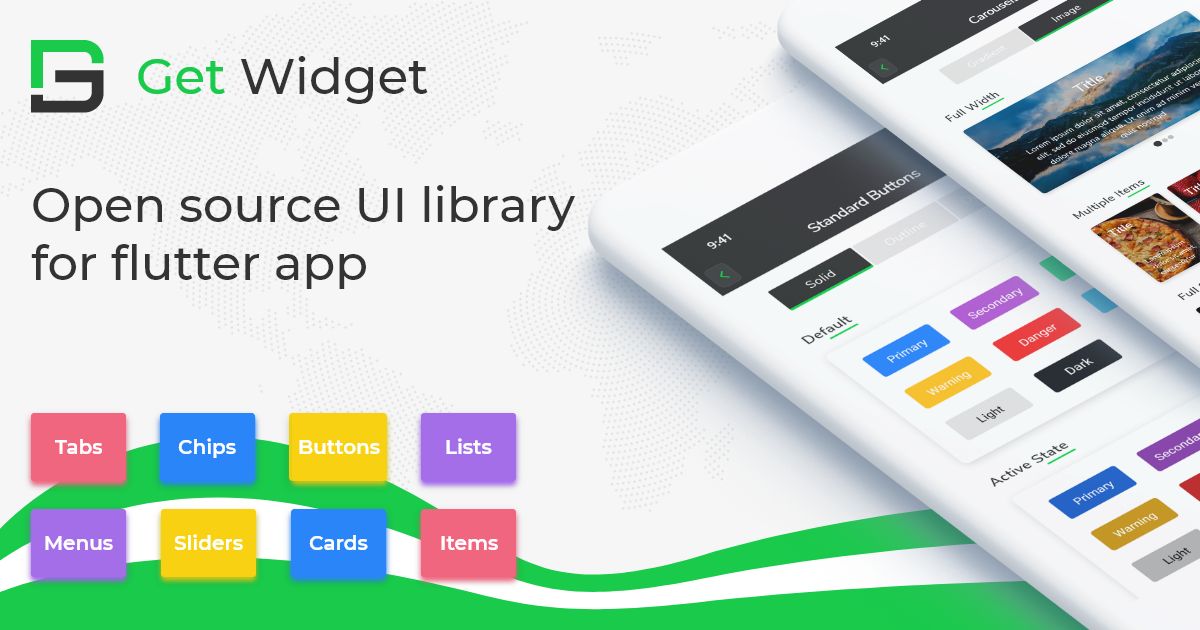
GitHub Repository: Please do appreciate our work through Github start
Also Read – Top 10 Best Flutter Search Bar Widgets List
Conclusion:
Here we discuss, what Flutter SearchBar Widget is? And how we can use and implement it into our Flutter app through the GetWidget SearchBar component. Here, there are options to customize the SearchBar.
About Our team
We have been working on Flutter since Flutter launched in beta version in 2017. And our team have been putting hundreds and hundreds of hour to experiment and implementation of Flutter. As well as after successful delivery of enterprise and SAAS applications that have been used by more than 500+ businesses around the 119+ countries. Now we are in love with Flutter development and we are very passionate about Flutter development. Now it is something we are trying to give a small contribution to the Flutter Dev Community.