A Flutter Bottom Sheet is a Flutter material widget that slides up from the bottom edge of the mobile screen and is an alternative to the modal or popups. It also can show different components by swiping up. The sheet hosts many other widgets inside it.
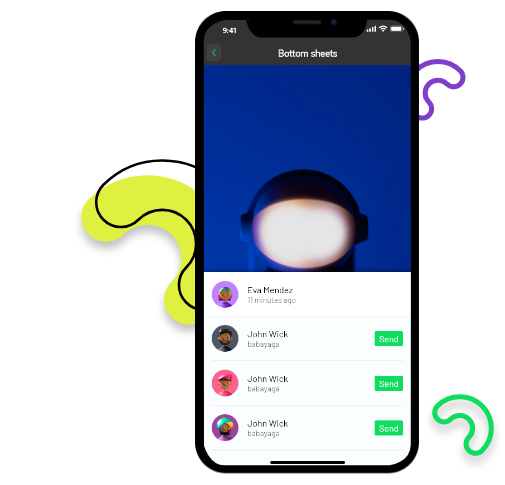
Flutter Bottom Sheet Modal
Flutter Bottom Sheet behavior can be applied to a child of Coordinator Layout to make that child a persistent bottom sheet. Persistent bottom sheets are views that come up from the bottom of the screen and overlap over the main widget. we can drag vertically to expose more or less of their content.
Now here is i am going to talk about what is GetWidget Bottom Sheet and how we implement this on Flutter app to build awesome Flutter Bottom Sheet for an app.
How to Start:
Now here is the guide about how we should start developing the GF Flutter Bottomsheet Widget with the use of the GetWidget UI Library.
Getwidget Getting Started will guide you on how to start building a beautiful flutter application with the GetWidget UI library. You have to install the package from pub.dev, and import the package in your Flutter project.
Install Package from pub.dev :
https://pub.dev/packages/getwidget
Import full package:
import ‘package:getwidget/getwidget.dart’;
Note: dependencies: getwidget: ^ 4.0.0
Keep playing with the pre-built UI components.
In the GFBottomSheet component, we have expandable content wherein we can expand the body or the content of the sheet according to our needs.
In the below section let us see how to use the sheet in the application with other widgets inside it.
How to create a Flutter Bottom Sheet widget?
Flutter Bottom Sheet is a material widget that is used as a modal/popup that slides from the bottom of the screen. When the bottom sheet is active, the other functions of the screen won’t be active to operate, in other words, it will be disabled until the bottom sheet is active on the screen.
Basic GFBottomsheet will slide up and down with a content body. The appearance of the GFBottomsheet can be customized using the GFBottomsheet properties.
Here, we will be using the GetWidget library and its GFBottomSheet component that has many customization options to use and make the bottom sheet as per the user requirement.
GFBottomSheet is a Flutter Bottom Sheet that is a material widget that popups itself from the floor of the screen. When the bottom sheet is opened the other functions of the respective screen will not work and be static.
Let us now see how the Flutter Bottom Sheet works with an example.
We can use the given example code to build the Flutter Bottom Sheet.
final GFBottomSheetController _controller = GFBottomSheetController();
Scaffold(
bottomSheet: GFBottomSheet(
controller: _controller,
maxContentHeight: 150,
stickyHeaderHeight: 100,
stickyHeader: Container(
decoration: BoxDecoration(color: Colors.white,
boxShadow: [BoxShadow(color: Colors.black45, blurRadius: 0)]
),
child: const GFListTile(
avatar: GFAvatar(
backgroundImage: AssetImage('assets image here'),
),
titleText: 'Title',
subtitleText: 'Sub Title goes here',
),
),
contentBody: Container(
height: 200,
margin: EdgeInsets.symmetric(horizontal: 15, vertical: 10),
child: ListView(
shrinkWrap: true,
physics: const ScrollPhysics(),
children: const [
Center(
child: Text(
'Lorem ipsum dolor sit amet, consectetur adipiscing elit, sed do eiusmod tempor incididunt ut labore et dolore magna aliqua. Ut enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillum dolore eu fugiat nulla pariatur. ',
style: TextStyle(
fontSize: 15, wordSpacing: 0.3, letterSpacing: 0.2),
))
],
),
),
stickyFooter: Container(
color: GFColors.SUCCESS,
child: Row(
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Text(
'Call us',
style: TextStyle(
fontSize: 18,
fontWeight: FontWeight.bold,
color: Colors.white),
),
Text(
'Email us',
style: TextStyle(fontSize: 15, color: Colors.white),
),
],
),
),
stickyFooterHeight: 50,
),
floatingActionButton: FloatingActionButton(
backgroundColor: GFColors.SUCCESS,
child: _controller.isBottomSheetOpened ? Icon(Icons.keyboard_arrow_down):Icon(Icons.keyboard_arrow_up),
onPressed: () {
_controller.isBottomSheetOpened
? _controller.hideBottomSheet()
: _controller.showBottomSheet();
}
),
)
Flutter Bottom Sheet Modal Example code
GF Flutter Bottom Sheet with Expandable Content
GF Bottomsheet allows users to expand the content body to display more content. The user can decide on how much the content should be expanded according to the requirement.
The below code shows how a bottom sheet in Flutter and its content can be expanded accordingly.
final GFBottomSheetController _controller = GFBottomSheetController();
GFBottomSheet(
controller: _controller,
maxContentHeight: 300,
enableExpandableContent: true,
stickyHeaderHeight: 100,
stickyHeader: Container(
decoration: BoxDecoration(
color: Colors.white,
boxShadow: [BoxShadow(color: Colors.black45, blurRadius: 0)]),
child: const GFListTile(
avatar: GFAvatar(
backgroundImage: AssetImage('asset image here'),
),
titleText: 'Charles Aly',
subtitleText: '20 minutes ago',
),
),
contentBody: SingleChildScrollView(
child: Container(
margin: EdgeInsets.symmetric(horizontal: 15, vertical: 10),
child: Column(
children: [
Container(
margin: EdgeInsets.symmetric(horizontal: 15),
child: Row(
children: [
Container(
width: 30,
height: 30,
decoration: BoxDecoration(
borderRadius: BorderRadius.circular(50),
image: DecorationImage(
image: AssetImage(
'asset image here'))),
),
Container(
margin: EdgeInsets.only(left: 6),
child: Text(
'Add to your story',
style: TextStyle(color: Colors.blue, fontSize: 15,
fontWeight: FontWeight.bold,),
))
],
),
),
ListView.builder(
physics: ScrollPhysics(),
shrinkWrap: true,
scrollDirection: Axis.vertical,
itemCount: 3,
itemBuilder: (BuildContext context, int index) {
return SingleChildScrollView(
child: InkWell(
child: GFListTile(
avatar: GFAvatar(
backgroundImage:
AssetImage('asset image here'),
size: 20,
),
subtitleText: 'Syam Aly',
icon: Container(
width: 66,
height: 30,
child: GFButton(
onPressed: () {},
color: GFColors.PRIMARY,
child: Center(
child: Text(
'Send',
style: TextStyle(color: Colors.white),
)),
),
),
),
));
}),
],
),
),
),
),
Flutter Bottomsheet with expanded content
The look and feel of the GFBottomsheet can be customized using the GFBottomsheet custom properties to make it look more attractive to the user.
Name | Description |
---|---|
minContentHeight | [minContentHeight] controls the minimum height of the content body. Content body with [minContentHeight] displays only when [enableExpandableContent] is false. It Must be greater or equal to 0. Default value is 0. |
maxContentHeight | [maxContentHeight] controls the maximum height of the content body. It Must be greater or equal to 0. Default value is 300. |
stickyHeader | [stickyHeader] is the header of GFBottomSheet. User can interact by swiping or tapping the [stickyHeader] |
contentBody | [contentBody] is the body of GFBottomSheet. User can interact by swiping or tapping the [contentBody] |
stickyFooter | [stickyFooter] is the footer of GFBottomSheet. User can interact by swiping or tapping the [stickyFooter] |
stickyFooterHeight | [stickyFooterHeight] defines the height of GFBottomSheet’s [stickyFooter] |
stickyHeaderHeight | [stickyHeaderHeight] defines the height of GFBottomSheet’s [stickyHeader] |
elevation | [elevation] controls shadow below the GFBottomSheet material. Must be greater or equal to 0. Default value is 0. |
enableExpandableContent | [enableExpandableContent] allows [contentBody] to expand. Default value is false. |
controller | [controller] used to control GFBottomSheet behavior like hide/show |
animationDuration | Defines the drag animation duration of the GFBottomSheet Default value is 300. |
What is a Persistent Bottom Sheet?
The Persistent Bottom Sheet is a Flutter BottomSheet that is just like the other widgets on the same screen. As the name itself tells, that it is a constant or continuous widget that goes hand in hand with the actual UI section on the present respective screen. It is an interaction between the main UI block and the menu dialog, which both constitute the Persistent Bottom Sheet.
What are the customizable properties used in Flutter Bottom Sheet?
The customizable properties include the min-content height, max-content height, sticky header, sticky footer, content body, elevation, animation duration, sticky header height, sticky footer height of the Bottom Sheet component. Hence customizable properties are fully flexible. You can check out all custom properties on GetWidget Bottom Sheet Widget Properties.
Can we expand the content body?
Yes, we can expand the content body by enabling the property enableExpandableContent
on true
.
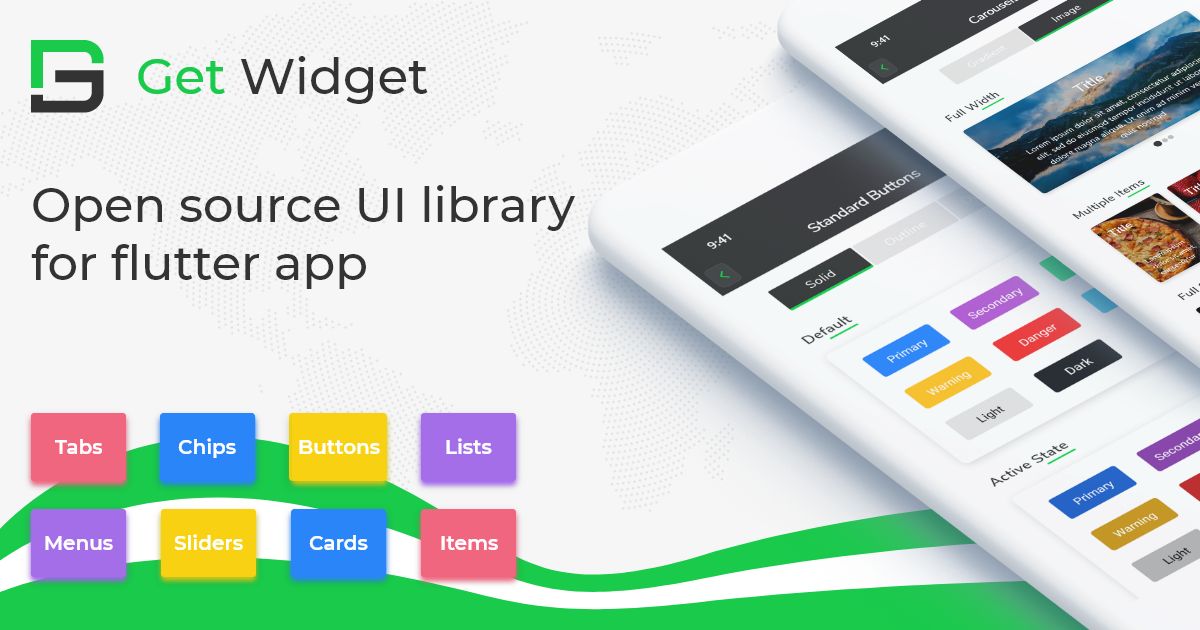
GitHub Repository: Please do appreciate our work through GitHub start
Conclusion:
Here we discussed, what Flutter GFBottomSheet Widget is. And how we can use and implement it into our Flutter app through the GetWidget GFBottomSheet component. Here, there are options to customize and use other types of GFBottomSheet
About Our Team:
We have been working on Flutter since Flutter launched in beta version in 2017. And our team has been putting hundreds and hundreds of hours into the experiment and implementation of Flutter. As well as After successfully delivering enterprise and SAAS applications that have been used by more than 500+ businesses around 119+ countries. Now we are in love with Flutter app development and we are very passionate about Flutter development. Now it is something we trying to make a small contribution to the Flutter Dev Community.