Flutter Animation is an abstract class that helps to understand the components’ current values and their states. we mostly used animation to create models that are required for research and study. Animation also allows us to create realistic models that help us to show the accurate representation of a component. Animations enhance the user experience that makes the applications more interactive for the users and user-friendly.

Flutter Animation Widget
Flutter uses an animation widget to make the component’s state dynamic. We can customize the animation by using its property. Nowadays animations are used to make their components more interactive.
In Flutter, we can define animation inside scaffold but before declaring animation we need to declare animation controller.
Flutter Animation Controller
A controller for animation. This class lets us perform tasks such as Play an animation forward or in reverse, or stop an animation, etc. Set the animation to a specific value.
By using different properties of the controller we can customize the animation duration, parent, and curve structure.
Also Read: How to Build Flutter Loader Widget
How to use custom animation with GFWidget
It allows to speed up overall Flutter app development by 20-30%. It has 1000+ widget component that allows you to enhance your Flutter UI design. And it will speed up in flutter development process with fully customizable features control.
Now here is i am going to talk about what is GetWidget Animation widget and how we implement this on Flutter app to build awesome Flutter Animation widget for an app.
How to Get Start with GFAnimation
Now here is the guide about how we should start developing GFAnimation Widget with the use of GetWidget UI Library.
Getting started will guide you how to start building a beautiful flutter application with GetWidget UI library. You have to install the package from pub.dev, import the package in your Flutter project.
Install Package from pub.dev :
https://pub.dev/packages/getwidget
Import full package:
import ‘package:getwidget/getwidget.dart’;
Note: dependencies: getwidget: ^ 4.0.0
Keep playing with the pre-built UI components.
Also Read: How to build Flutter Auto Complete Custom Searchbar
Flutter Rotation Animation
Rotation GFAnimation is a Flutter Rotation Animation widget that will rotate the component. GFAnimation property type: GFAnimationType.rotateTransition
creates a rotation transition for the child of a widget. We can use the below code to create a rotation animation for a component.

import 'package:getwidget/getwidget.dart';
AnimationController controller;
Animation animation;
@override
void initState() {
super.initState();
controller =
AnimationController(duration: const Duration(seconds: 10), vsync: this);
animation = new CurvedAnimation(parent: controller, curve: Curves.linear);
controller.repeat();
}
GestureDetector(
onTap: () {
controller.repeat();
},
child: GFAnimation(
turnsAnimation: animation,
controller: controller,
type: GFAnimationType.rotateTransition,
alignment: Alignment.center,
child: Image.asset(
'assets image here',
width: 100,
height: 120,
),
),
),
Flutter Scaling Animation
Flutter Scaling GFAnimation apparently changes the size of a widget from smaller to bigger or vice versa. GFAnimation property type: GFAnimationType.scaleTransition
. Creates a scale transition for the child of the widget. We can use the below code for scaling animation.

import 'package:getwidget/getwidget.dart';
AnimationController controller;
Animation animation;
@override
void initState() {
super.initState();
controller =
AnimationController(duration: const Duration(seconds: 10), vsync: this);
animation = new CurvedAnimation(parent: controller, curve: Curves.linear);
controller.repeat();
}
GestureDetector(
onTap: () {
controller.repeat();
},
child: GFAnimation(
scaleAnimation: animation,
controller: controller,
type: GFAnimationType.scaleTransition,
child: Image.asset(
'assets image here',
width: 110,
height: 90,
),
),
),
Flutter Alignment Animation
Flutter Alignment GFAnimation aligns your component horizontally or else vertically. If you are giving a particular alignment then with the given duration it will align your component to the targeted alignment position. GFAnimation property type: GFAnimationType.align
, creates alignment transitions for the child of the widget. We can use the below code for alignment animation.

import 'package:getwidget/getwidget.dart';
GFAnimation(
duration: Duration(seconds: 4),
alignment: Alignment.bottomLeft,
type: GFAnimationType.align,
child: Image.asset(
'assets image here',
width: 130,
height: 130,
),
),
Flutter Slide Transition With GFAnimation
Slide Transition GFAnimation animates the widget from one position to another relative position. GFAnimation property type: GFAnimationType.slideTransition
, Creates a fractional translation transition for the child of the widget. We can use the below code for slide transition animation.

import 'package:getwidget/getwidget.dart';
Animation offsetAnimation;
@override
void initState() {
super.initState();
controller =
AnimationController(duration: const Duration(seconds: 6), vsync: this);
animation = new CurvedAnimation(parent: controller, curve: Curves.linear);
controller.repeat();
offsetAnimation = Tween(
begin: Offset(-5, 0),
end: const Offset(0, 0),
).animate(CurvedAnimation(
parent: controller,
curve: Curves.linear,
));
}
Container(
width: MediaQuery.of(context).size.width,
child: GFAnimation(
controller: controller,
slidePosition: offsetAnimation,
type: GFAnimationType.slideTransition,
child: Image.asset(
'assets image here',
width: 110,
height: 110,
),
),
),
Flutter Size Animation
Flutter Size GFAnimation animates the widget that automatically transitions its size in a given duration whenever the given child’s size changes. GFAnimation property type: GFAnimationType.size
, creates a widget that animates its size. We can use the below code for size animation.

import 'package:getwidget/getwidget.dart';
bool selected = false;
AnimationController controller;
Animation animation;
@override
void initState() {
super.initState();
controller =
AnimationController(duration: const Duration(seconds: 7), vsync: this);
animation = new CurvedAnimation(parent: controller, curve: Curves.linear);
controller.repeat();
}
GFAnimation(
onTap: () {
if (mounted) {
setState(() {
selected = !selected;
});
}
},
width: selected ? 150 : 100,
height: selected ? 150 : 100,
type: GFAnimationType.size,
controller: controller,
child: Image.asset(
'assets image here',
width: 90,
height: 90,
),
),
Flutter Container Animation
Container GFAnimation animates the component that automatically transits its size in a given duration whenever the given child’s size changes. GFAnimation property type: GFAnimationType.container
, creates a widget that changes its size.

We can use the below code for container animation.
import 'package:getwidget/getwidget.dart';
AnimationController controller;
Animation animation;
double _fontSize = 40;
@override
void initState() {
super.initState();
controller =
AnimationController(duration: const Duration(seconds: 10), vsync: this);
animation = new CurvedAnimation(parent: controller, curve: Curves.linear);
controller.repeat();
}
GFAnimation(
width: 80,
changedWidth: 120,
height: 60,
changedHeight: 130,
activeColor: Colors.transparent,
color: Colors.transparent,
fontSize: _fontSize,
type: GFAnimationType.container,
child: Image.asset(
'assets image here',
width: 90,
height: 90,
),
),
What are the customizable properties used?
Ans. The customizable properties include the duration, reverseDuration, alignment, activeAlignment, child, curve, type, width, turnsAnimation, scaleAnimation, slidePosition, controller, onTap, changedHeight etc. of Drawer component . Hence customizable properties are full flexible. You can check out all custom properties on GetWidget Flutter Animation Widget Custom Properties.
Can our slide transition will work without offsetAnimation?
Ans: No, it will not work without a declaration of offsetAnimation. To make it work we have to declare the offsetAnimation inside scaffold because it holds the begin and end transition controllers which helps the components to detect the slidePosition.
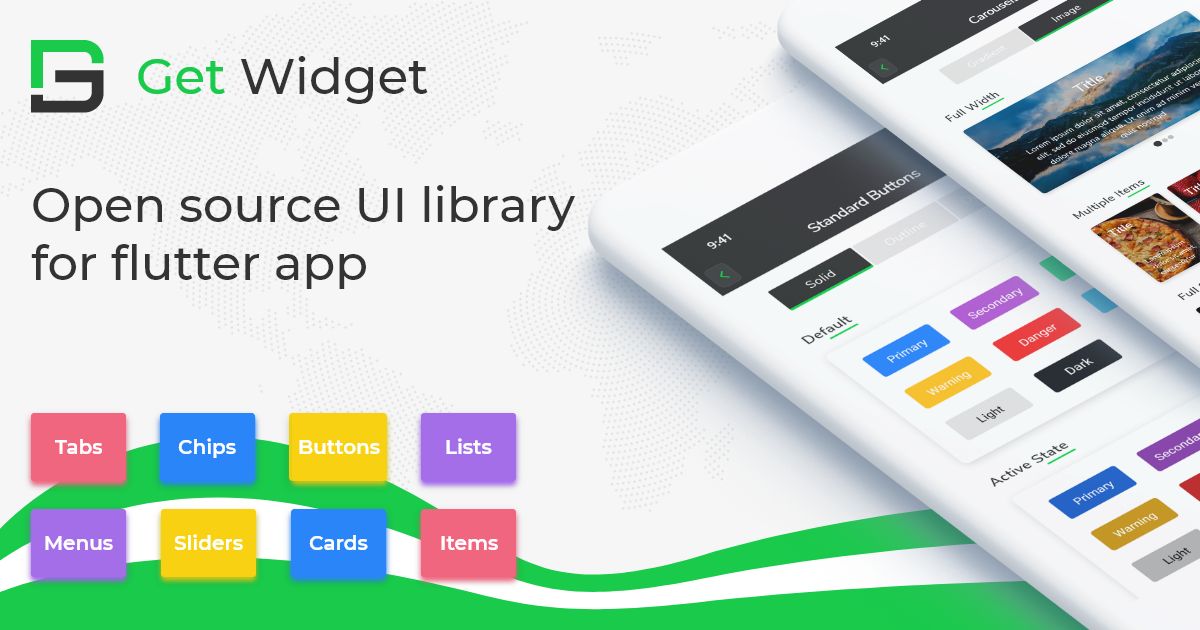
GitHub Repository: Please do appreciate our work through Github start
Conclusion:
Here we discuss, what Flutter Animation Widget is? And how we can use and implement it into our Flutter app through the GetWidget Animation component. Here, there are options to customize the Animation and the different Animation types that can be used.
About Our Team:
We have been working on Flutter since Flutter launched in beta version in 2017. And our team have been putting hundreds and hundreds of hour to experiment and implementation of Flutter. As well as After successfully delivering enterprise and SAAS applications that have been used by more than 500+ businesses around 119+ countries. Now we are in love with Flutter development and we are very passionate about Flutter development. Now it is something we trying to give a small contribution to the Flutter Dev Community.