Flutter Dropdown Widget
Flutter Dropdown List allows a user to select a particular item from several items which will be in a dropdown fashion. The default value can be an item inside the dropdown or any value the user needs. It has an arrow icon on the list in Flutter or the right upon clicking opens a list of items in which the user can select the desired option. It allows users to select one unique value from a set of values.
So, are you ready to make use of this widget package in the Flutter application? This Flutter widget performs auto search action after user action is performed. If so, then let’s quickly jump into the usage and the ways a Dropdown can be modified and used to make user-friendly apps. Here I am going to use an open-source UI Library known as GetWidget to build this Dropdown List widget in Flutter.
Flutter DropDown Widget
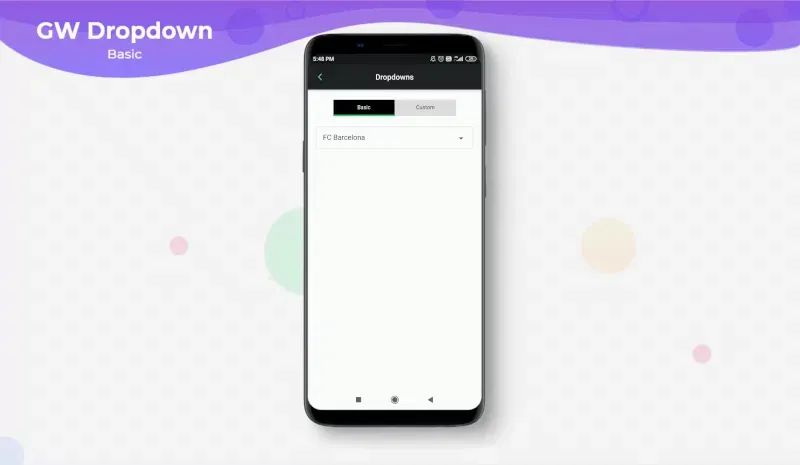
GetWidget Dropdown Widget Component is a component that allows users to select a unique value from a set of values which will be displayed upon clicking the arrow/dropdown box. It is useful in selecting a value for any kind of application. It has customization options which is an easy way to handle it as per the application and its requirement. The GetWidget Dropdown displays the list of items in an overlay dropdown fashion.
GetWidget Dropdown components can be classified as Single-select dropdown and Multi-Select Flutter dropdown. Let’s dive deep into the two types and see how it is used.
How to Start:
Now, here is the guide about how we should you can start developing the Flutter Dropdown Widget with the help of the GetWidget UI Library.
First, Let go with Getting started, It will guide you on how to start building a beautiful flutter application UI with the GetWidget UI library. Now, we have to install the GetWidget package from the pub.dev and use our 1000+ inbuilt Widget. To import the package in your Flutter project follow the below guide.
Install Package from pub.dev :
https://pub.dev/packages/getwidget – Check the details about GetWidget Package.
Import full package:
import ‘package:getwidget/getwidget.dart’;
Note: dependencies: getwidget: ^4.0.0
Keep playing with the pre-built UI components.
GFSingle-Select Flutter Dropdown
The single-select dropdown button simply selects a unique value from a set of values given in the dropdown. It displays the list of items in an overlay dropdown fashion.
The below code shows a simple single dropdown code that displays the single value from a list of options.
String dropdown;
Container(
height: 50,
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.all(20),
child: DropdownButtonHideUnderline(
child: GFDropdown(
padding: const EdgeInsets.all(15),
borderRadius: BorderRadius.circular(5),
border: const BorderSide(
color: Colors.black12, width: 1),
dropdownButtonColor: Colors.white,
value: dropdownValue,
onChanged: (newValue) {
setState(() {
dropdownValue = newValue;
});
},
items: [
'UI',
'Devops',
'API',
'Apps'
]
.map((value) => DropdownMenuItem(
value: value,
child: Text(value),
))
.toList(),
),
),
),
Flutter Dropdown Single Select Widget code example
The below code shows a customized simple single dropdown code that displays the single value from a list of options.
String dropdown;
Container(
height: 50,
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.all(20),
child: DropdownButtonHideUnderline(
child: GFDropdown(
padding: const EdgeInsets.all(15),
borderRadius: BorderRadius.circular(10),
border: const BorderSide(
color: Colors.black12, width: 1),
dropdownButtonColor: Colors.grey[300],
value: dropdownValue,
onChanged: (newValue) {
setState(() {
dropdownValue = newValue;
});
},
items: [
'UI',
'Devops',
'API',
'Apps'
]
// return DropdownMenuItem
.map((value) => DropdownMenuItem(
value: value,
child: Text(value),
))
.toList(),
),
),
),
Flutter Dropdown Single Select Widget Custom example code
GFMulti-Select Dropdown for Flutter App
Muti-select dropdown is used to select multiple values from a given set of checkbox items and display selected items in the TitleTile box. It displays the list of items in an overlay dropdown fashion.
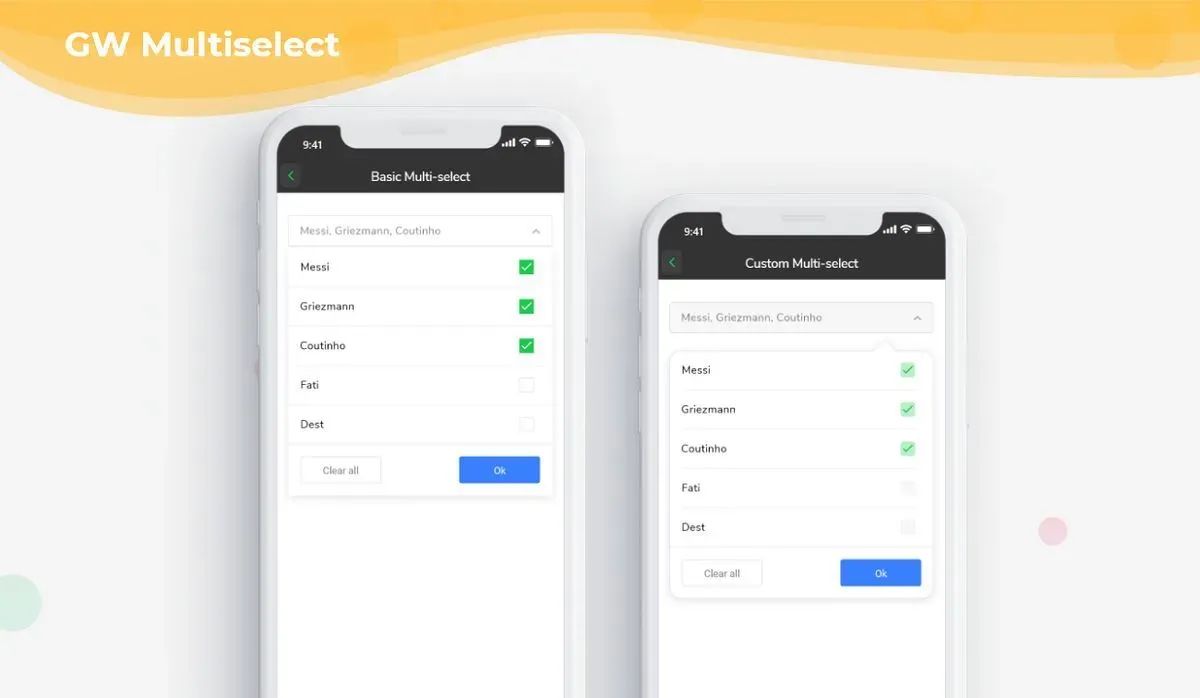
The below code shows a simple multi-select dropdown code that displays more than one value from a list of options.
GFMultiSelect(
items: dropList,
onSelect: (value) {
print('selected $value ');
},
dropdownTitleTileText: 'UI, API, Apps ',
dropdownTitleTileMargin: EdgeInsets.only(
top: 22, left: 18, right: 18, bottom: 5),
dropdownTitleTilePadding: EdgeInsets.all(10),
dropdownUnderlineBorder:
const BorderSide(color: Colors.transparent, width: 2),
dropdownTitleTileBorder:
Border.all(color: Colors.grey[300], width: 1),
dropdownTitleTileBorderRadius: BorderRadius.circular(5),
expandedIcon: const Icon(
Icons.keyboard_arrow_down,
color: Colors.black54,
),
collapsedIcon: const Icon(
Icons.keyboard_arrow_up,
color: Colors.black54,
),
submitButton: Text('OK'),
cancelButton: Text('Cancel'),
dropdownTitleTileTextStyle:
const TextStyle(fontSize: 14, color: Colors.black54),
padding: const EdgeInsets.all(6),
margin: const EdgeInsets.all(6),
type: GFCheckboxType.basic,
activeBgColor: GFColors.SUCCESS,
activeBorderColor: GFColors.SUCCESS,
inactiveBorderColor: Colors.grey[300],
),
Flutter Dropdown Multi-Select Widget example code
The below code shows a customized simple multi-select dropdown code that displays more than one value from a list of options.
Container(
child: GFMultiSelect(
items: dropList,
onSelect: (value) {
print('selected $value ');
},
dropdownTitleTileText: 'UI, API, Apps ',
dropdownTitleTileColor: Colors.grey[200],
dropdownTitleTileMargin: EdgeInsets.only(
top: 22, left: 18, right: 18, bottom: 5),
dropdownTitleTilePadding: EdgeInsets.all(10),
dropdownUnderlineBorder: const BorderSide(
color: Colors.transparent, width: 2),
dropdownTitleTileBorder:
Border.all(color: Colors.grey[300], width: 1),
dropdownTitleTileBorderRadius: BorderRadius.circular(5),
expandedIcon: const Icon(
Icons.keyboard_arrow_down,
color: Colors.black54,
),
collapsedIcon: const Icon(
Icons.keyboard_arrow_up,
color: Colors.black54,
),
submitButton: Text('OK'),
dropdownTitleTileTextStyle: const TextStyle(
fontSize: 14, color: Colors.black54),
padding: const EdgeInsets.all(6),
margin: const EdgeInsets.all(6),
type: GFCheckboxType.basic,
activeBgColor: Colors.green.withOpacity(0.5),
inactiveBorderColor: Colors.grey[200],
),
),
Flutter Dropdown Multi-Select Widget example custom code
The look and feel of the Flutter Singleselect DropdownEvents can be customized using the GFDropdown properties.
icon | The widget to use for the drop-down button’s icon. Defaults to an [Icon] with the [Icons.arrow_drop_down] glyph. |
elevation | The z-coordinate at which to place the menu when open. |
value | The value of the currently selected [DropdownMenuItem]. |
border | Defines the border of dropdown button |
padding | Defines the padding given inside the dropdown |
hint | A placeholder widget that is displayed by the dropdown button. |
disabledHint | A message to show when the dropdown is disabled. |
onChanged | Called when the user selects an item. If the [onChanged] callback is null or the list of [DropdownButton.items] is null then the dropdown button will be disabled, |
onTap | Called when the dropdown button is tapped. |
style | Defaults to the [TextTheme.subtitle1] value of the current [ThemeData.textTheme] of the current [Theme]. |
underline | The widget to use for drawing the drop-down button’s underline. |
iconDisabledColor | The color of any [Icon] descendant of [icon] if this button is disabled, i.e. if [onChanged] is null. |
iconEnabledColor | The color of any [Icon] descendant of [icon] if this button is enabled, i.e. if [onChanged] is defined. |
iconSize | The size to use for the drop-down button’s down arrow icon button. |
isDense | Reduce the button’s height. |
isExpanded | Set the dropdown’s inner contents to horizontally fill its parent. |
itemHeight | defines the height of the menu items |
focusColor | The color for the button’s [Material] when it has the input focus. |
dropdownColor | Defines the background color of the dropdown. |
borderRadius | Defines the border radius of the dropdown. |
dropdownButtonColor | Defines the background color of the dropdownButton. |
autofocus | On true state it should focus itself if nothing else is already focused. Defaults to false |
focusNode | Defines the keyboard focus for this widget. |
The look and feel of the Multiselect Dropdown list widget can be customized using the GFMultiselect properties.
items | defines the list of items the user can select |
onSelect | callback when user select item from the dropdown, in callback we get list of selected items index |
dropdownTitleTileText | type of [String] to define the dropdownTitleTile title |
dropdownTitleTileTextStyle | type of [TextStyle] to define the textStyle of [dropDownTitleTileText] |
dropdownTitleTileHintText | type of [String] to define the dropdownTitleTile hint text |
dropdownTitleTileHintTextStyle | type of [TextStyle] to define the textStyle of [dropDownTitleTileHintTextStyle] |
dropdownTitleTileBorderRadius | defines the border radius of the dropdownTitleTile |
dropdownTitleTileBorder | defines the border of the dropdownTitleTile. |
dropdownTitleTileColor | defines the background color of dropdownButton |
hideDropdownUnderline | on true state, it hides the Dropdown Underline border defaults value is false |
dropdownUnderlineBorder | defines the border of the Dropdown Underline border |
dropdownTitleTileMargin | defines the dropdownTitleTile margin |
dropdownTitleTilePadding | defines the dropdownTitleTile padding |
expandedIcon | defines the dropdownTitleTile’s trailing icon when dropdown is visible |
collapsedIcon | defines the dropdownTitleTile’s trailing icon when dropdown is not visible |
submitButton | defines the button in the dropdown |
color | defines dropdown checkbox ListTile’s background color. Can be given [Color] or [GFColors] |
avatar | type of [Widget] or [GFAvatar] used to defines dropdown checkbox ListTile’s leading |
margin | defines the margin of dropdown checkbox ListTile |
padding | defines the padding of dropdown checkbox ListTile |
type | defines dropdown ListTile’s checkbox type type of [GFCheckboxType] which is of four type is basic, sqaure, circular and custom |
size | defines dropdown ListTile’s checkbox size type of [double] which is GFSize ie, small, medium and large and can use any double value |
activeBgColor | defines dropdown ListTile’s checkbox background color when its active type of [Color] used to change the backgroundColor of the active checkbox |
inactiveBgColor | defines dropdown ListTile’s checkbox background color when its inactive type of [Color] used to change the backgroundColor of the inactive checkbox |
activeBorderColor | defines dropdown ListTile’s checkbox border color when its active type of [Color] used to change the border color of the active checkbox |
inactiveBorderColor | defines dropdown ListTile’s checkbox border color when its inactive type of [Color] used to change the border color of the inactive checkbox |
activeIcon | defines dropdown ListTile’s checkbox’s active icon type of [Widget] used to change the checkbox’s active icon |
inactiveIcon | defines dropdown ListTile’s checkbox’s inactive icon type of [Widget] used to change the checkbox’s inactive icon |
customBgColor | type of [Color] used to change the background color of the custom active checkbox only |
selected | To have the list tile appear selected when the checkbox is checked, pass the same value to both. Normally, this property is left to its default value, false. |
dropdownBgColor | defines the background color of the dropdown. Can be given [Color] or [GFColors] |
Can we change the arrow button?
Yes, it can be customized according to the need.
Can we customize the checkbox list used in the multi-select dropdown?
Yes, it is fully customized as we are using GFCheckboxListTile and it has many options to customize as per the needs.
Where was Typically Dropdown Button Widget used?
This widget is typically used in the Item search feature.
How to create a Flutter Dropdown
The flutter dropdown Button is a material widget that is used to display the items or list in a dropdown fashion. The user is able to select any one of the items from the list given in the form of the dropdown. The dropdown is used when there are multiple items and saves a lot of space where the lists will be collapsed under the dropdown button.
The dropdown button has an arrow to indicate that the items in the list are not expanded and when the whole button along the arrow is clicked the dropdown list item opens. When the item is selected it will appear on the space provided by the button.
Here to demonstrate the example of the dropdown we will be using the Dropdown of the GetWidget library.
In GetWidget library Dropdown is divided into Single-item dropdown and multi-select dropdown. Let us see one by one in the below sections.
Single-Selection Dropdown option in Flutter
Single-select dropdown selects a unique and only one value from a set of values given in the dropdown. It displays the list of items in an overlay dropdown fashion.
The below code shows a simple single dropdown code that displays the single value from a list of options.
Multi-Select Dropdown in Flutter
Multi-select dropdown is used in selecting more than one item or in other words selecting multiple values from a given set of checkbox items and display selected items in the TitleTile box. It displays the list of items in an overlay dropdown fashion.
The below code shows a simple multi-select dropdown code that displays more than one value from a list of options.
The above examples of both types of dropdowns can be customized according to the need. Head to the official documentation of GetWidget to know more about the components.
How to create a Custom Flutter Dropdown
Dropdown in Flutter is a button that has a list of items upon clicking can be selected and the selected item is displayed on the button. The dropdown is useful where there are many options and the selection has to be made between the given options.
The dropdown button has an arrow in it to show the dropdown list items and upon clicking the arrow or the button, the list of items opens, on which the user can select the required option.
The GetWidget library has a Dropdown Component which we will be using here to demonstrate the example of the dropdown. Dropdown in Getwidget can be classified into Single and Multi-level dropdowns.
let us go one by one in the below sections.
GFSingle-Select Flutter Dropdown:
Single-select dropdown selects a unique value from a set of values given in the dropdown. It displays the list of items in an overlay dropdown fashion.
The below code shows a simple single dropdown button code that displays the single value from a list of options.
String dropdown;
Container(
height: 50,
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.all(10),
child: DropdownButtonHideUnderline(
child: GFDropdown(
padding: const EdgeInsets.all(5),
borderRadius: BorderRadius.circular(15),
border: const BorderSide(
color: Colors.black12, width: 1),
dropdownButtonColor: Colors.white,
value: dropdownValue,
onChanged: (newValue) {
setState(() {
dropdownValue = newValue;
});
},
items: [
'UI',
'Devops',
'API',
'Apps'
]
.map((value) => DropdownMenuItem(
value: value,
child: Text(value),
))
.toList(),
),
),
),
Flutter Dropdown Single option selection example with GFDropdown
The below code shows a customized simple single dropdown code that displays the single value from a list of options.
String dropdown;
Container(
height: 50,
width: MediaQuery.of(context).size.width,
margin: EdgeInsets.all(5),
child: DropdownButtonHideUnderline(
child: GFDropdown(
padding: const EdgeInsets.all(5),
borderRadius: BorderRadius.circular(15),
border: const BorderSide(
color: Colors.black12, width: 1),
dropdownButtonColor: Colors.grey[300],
value: dropdownValue,
onChanged: (newValue) {
setState(() {
dropdownValue = newValue;
});
},
items: [
'UI',
'Devops',
'API',
'Apps'
]
// return DropdownMenuItem
.map((value) => DropdownMenuItem(
value: value,
child: Text(value),
))
.toList(),
),
),
),
Flutter Dropdown Single Custom option selection example with GFDropdown
GFMulti-Select Flutter Dropdown
Muti-select dropdown is used to select multiple values from a given set of checkbox items and display selected items in the TitleTile box. It displays the list of items in an overlay dropdown fashion.
The below code shows a simple multi-select dropdown code that displays more than one value from a list of options.
GFMultiSelect(
items: dropList,
onSelect: (value) {
print('selected $value ');
},
dropdownTitleTileText: 'UI, API, Apps ',
dropdownTitleTileMargin: EdgeInsets.only(
top: 22, left: 18, right: 18, bottom: 5),
dropdownTitleTilePadding: EdgeInsets.all(10),
dropdownUnderlineBorder:
const BorderSide(color: Colors.transparent, width: 2),
dropdownTitleTileBorder:
Border.all(color: Colors.grey[300], width: 1),
dropdownTitleTileBorderRadius: BorderRadius.circular(5),
expandedIcon: const Icon(
Icons.keyboard_arrow_down,
color: Colors.black54,
),
collapsedIcon: const Icon(
Icons.keyboard_arrow_up,
color: Colors.black54,
),
submitButton: Text('Submit'),
cancelButton: Text('Cancel'),
dropdownTitleTileTextStyle:
const TextStyle(fontSize: 14, color: Colors.black54),
padding: const EdgeInsets.all(6),
margin: const EdgeInsets.all(6),
type: GFCheckboxType.basic,
activeBgColor: GFColors.DANGER,
activeBorderColor: GFColors.DANGER,
inactiveBorderColor: Colors.grey[400],
),
Flutter Dropdown Multi-Select Custom option example with GFDropdown
The below code shows a customized simple multi-select dropdown code that displays more than one value from a list of options.
Container(
child: GFMultiSelect(
items: dropList,
onSelect: (value) {
print('selected $value ');
},
dropdownTitleTileText: 'UI, API, Apps ',
dropdownTitleTileColor: Colors.grey[200],
dropdownTitleTileMargin: EdgeInsets.only(
top: 22, left: 18, right: 18, bottom: 5),
dropdownTitleTilePadding: EdgeInsets.all(10),
dropdownUnderlineBorder: const BorderSide(
color: Colors.transparent, width: 2),
dropdownTitleTileBorder:
Border.all(color: Colors.grey[300], width: 1),
dropdownTitleTileBorderRadius: BorderRadius.circular(5),
expandedIcon: const Icon(
Icons.keyboard_arrow_down,
color: Colors.black54,
),
collapsedIcon: const Icon(
Icons.keyboard_arrow_up,
color: Colors.black54,
),
submitButton: Text('OK'),
dropdownTitleTileTextStyle: const TextStyle(
fontSize: 14, color: Colors.black54),
padding: const EdgeInsets.all(6),
margin: const EdgeInsets.all(6),
type: GFCheckboxType.basic,
activeBgColor: Colors.green.withOpacity(0.5),
inactiveBorderColor: Colors.grey[200],
),
),
Flutter Dropdown Multi-Select Custom option example with GFDropdown
The above examples of dropdowns can be customized according to the need of the user and the application. Head to the official documentation of GetWidget to know more about the components.
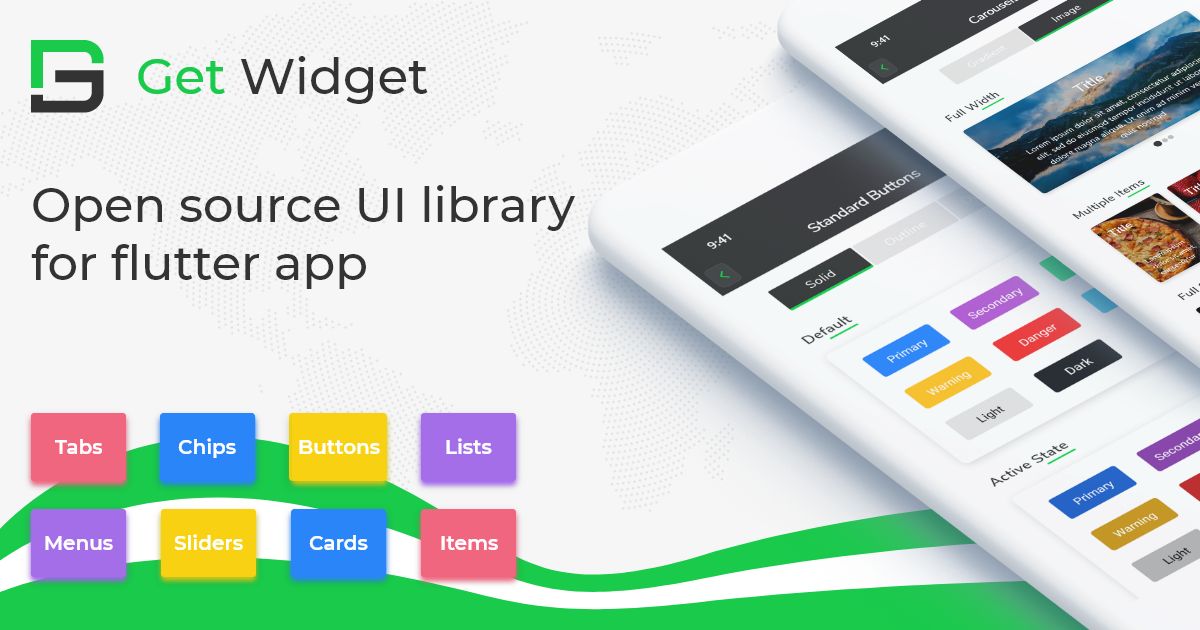
GitHub Repository: Please do appreciate our work through Github start
Conclusion:
Here we discussed, what Flutter Dropdown Widget is? And how it is easy and powerful to use in any application through the GetWidget Dropdown component for Flutter apps. Here, there are options to customize and use other types of dropdowns. Or hire flutter developer for creating a Flutter dropdown widget.